How to Make Gravity in Scratch How to Make Gravity in Scratch Easy
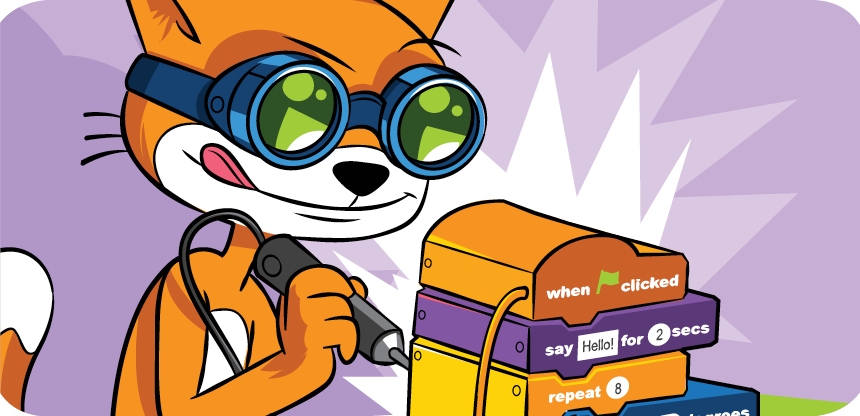
4
Shooting Hoops with Gravity
any platformer games, such as Super Mario Bros. and Sonic the Hedgehog, show the action from the side, with the ground at the bottom of the screen and the character appearing in profile. These games have gravity: characters can jump up and then drop until they land on the ground. In this chapter, we'll write a basketball game that has gravity. The player will jump and throw a basketball, and the basketball player and ball will drop to the ground.
Before you start coding, look at the final program at https://nostarch.com/scratch3playground/.
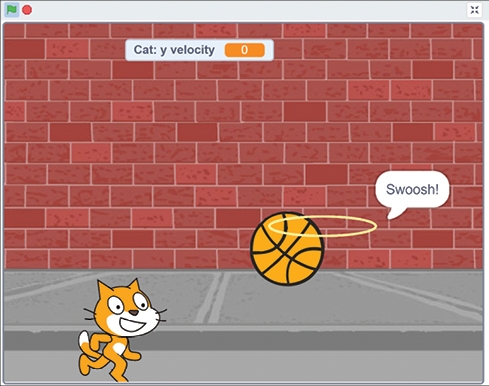
Sketch Out the Design
First, let's sketch out what we want the game to do. The player controls the cat, which can move left and right and jump. The goal is to have the cat shoot baskets into a moving basketball hoop, which will glide around the Stage randomly.
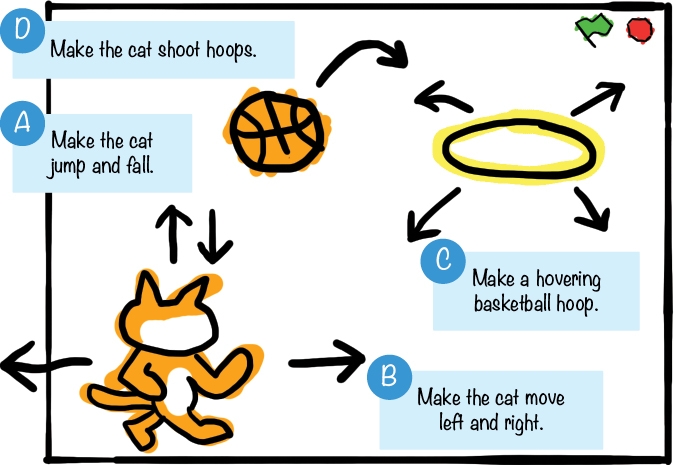
If you want to save time, you can start from the skeleton project file, named basketball-skeleton.sb3, in the resources ZIP file. Go to https://nostarch.com/scratch3playground/ and download the ZIP file to your computer by right-clicking the link and selecting Save link as or Save target as. Extract all the files from the ZIP file. The skeleton project file has all the sprites already loaded, so you'll only need to drag the code blocks into each sprite.
Make the Cat Jump and Fall
Let's start by adding gravity to make the cat jump up and come back down.
1. Add the Gravity Code to the Cat Sprite
Rename the Sprite1
sprite to Cat
in the Sprite List. Then rename the program from Untitled to Basketball in the text field at the top of the Scratch editor.
Click the Choose a Backdrop button in the lower right to open the Backdrop Library window. Select Wall 1 to change the backdrop. The Stage will look like this:
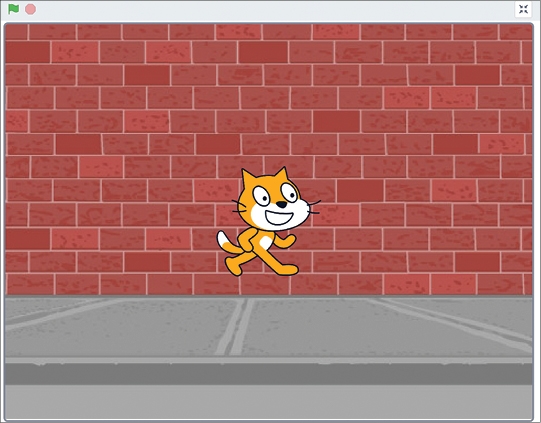
Programming gravity requires variables. You can think of a variable as a box for storing numbers or text that you can use later in your program. We'll create a variable that contains a number representing how fast the cat is falling.
First, make sure the Cat
sprite is selected in the Sprite List; then click the Scripts tab. In the orange Variables category, click the Make a Variable button to bring up the New Variable window. Enter y
velocity
as the variable name. (Velocity means how fast something is going and in what direction. When y
velocity
is a positive number, the cat moves up. When y
velocity
is a negative number, the cat moves down.) Make sure For this sprite only is selected. (If you see only For all sprites, the Stage is selected, not the Cat
sprite.) Then click OK.
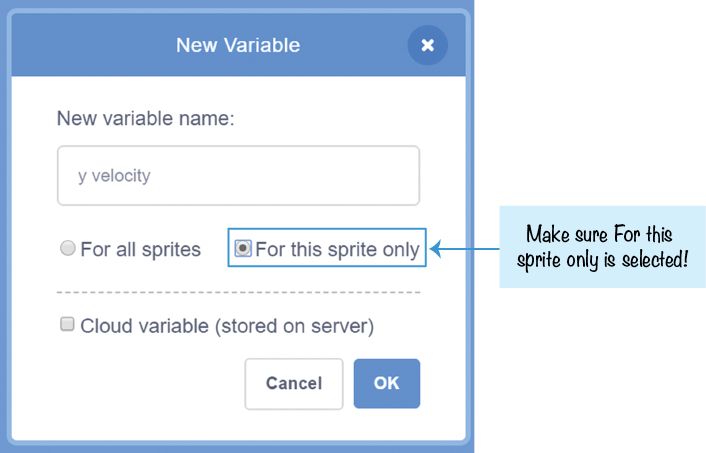
Several new blocks will appear in the Variables category, and one of those is the round y
velocity
variable block.
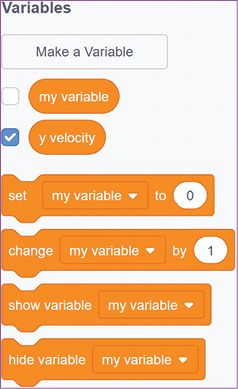
To put a value in your variable, you use the orange set
to
block. For example, if you created a variable greeting
, you could use set
to
to put the value Hello!
in it. Then, you could use greeting
in a say
block, which would be the same as entering Hello!
. (Don't add these blocks or create a greeting
variable in your Basketball program; this is just an example.)
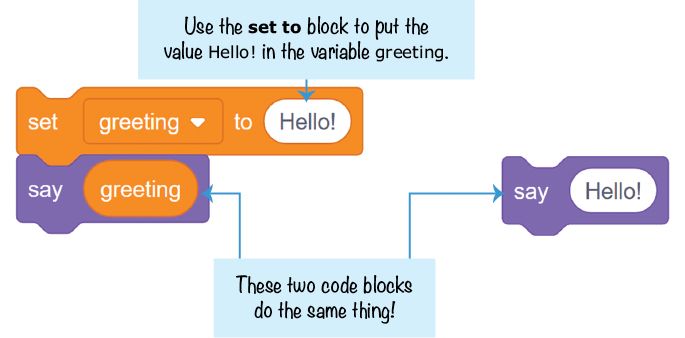
Using the variable lets the say
block change its text while the program runs. If you want to change the greeting text while the program is running, you can add another set
to
block to your program. If the variable contains a number, you can add to or subtract from this number by using the change
by
block.
Gravity makes objects accelerate downward. In the game, the Cat
sprite has to move down, and the speed at which it moves down must change while the program is running. Add the following code to the Cat
sprite to add gravity to your Scratch program. This is the minimal amount of code you need to make a sprite fall under gravity. You could add this code to any sprite to make it fall.
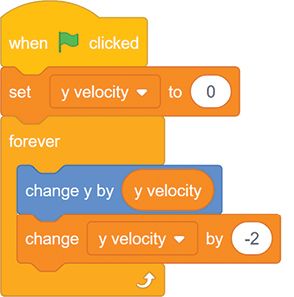
When you click the green flag, the y
velocity
variable is set to 0
, and the script enters a forever
loop. The y position (vertical position) of the Cat
sprite is changed by y
velocity
, and y
velocity
is changed by -2
. As the program goes through the loop, the y position will change faster and faster, making the cat fall faster and faster.
2. Add the Ground-Level Code
Right now, the cat falls. But we want the cat to stop when it hits the ground. Let's keep adding to the Cat
sprite's code so it looks this:
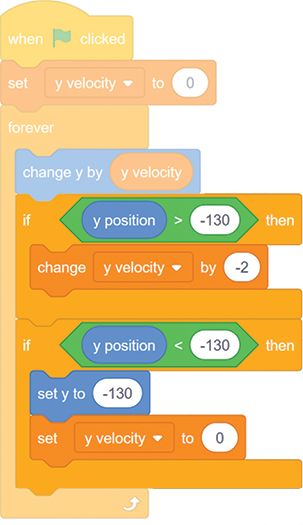
In this code, we set the y
position
of the ground level to -130
. If the Cat
sprite's y position is greater than (above) the ground level, then y
velocity
is changed by -2
, and the Cat
sprite will fall. Eventually, the Cat
sprite will fall past -130
, and its y position will be less than (below) the -130
ground level. When that happens, the Cat
sprite will be reset at the ground level of -130
, and y
velocity
will go back to 0
to stop the sprite from falling.
When you're done testing your program, make the y
velocity
variable invisible on the Stage by unchecking the checkbox next to it in the orange Data category.
3. Add the Jumping Code to the Cat Sprite
After adding the gravity code to the Cat
sprite, making the cat jump is easy. Add this code to the Cat
sprite:
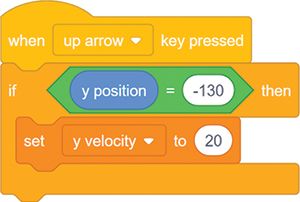
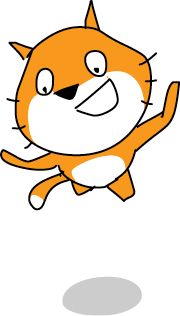
Now when you press the up arrow key, y
velocity
is set to the positive number 20
, making the Cat
sprite jump up. But the y
velocity
variable will still be changed by -2
each time the loop runs. So although the cat jumps by 20 at first, the next time through the loop, it will be at 18, then 16, and so on. Notice that the if
then
block checks that the Cat
sprite is on the ground. You shouldn't be able to make the cat jump if it's already in midair!
When y
velocity
is set to 0
, the Cat
sprite is at the peak of the jump. Then y
velocity
changes by -2
each time through the loop, and the Cat
sprite continues falling until it hits the ground. Try experimenting with different numbers for the set
y
velocity
to
and change
y
velocity
by
blocks. Figure out how to make the cat jump higher or lower (but always above the ground) or make gravity stronger or weaker.
Make the Cat Move Left and Right
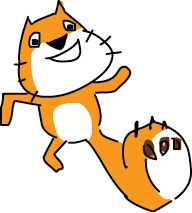
Let's add the Cat
sprite's walking code next so that the player can control the cat with the keyboard.
4. Add the Walking Code to the Cat Sprite
Add the following code to the bottom of the Cat
sprite's code:
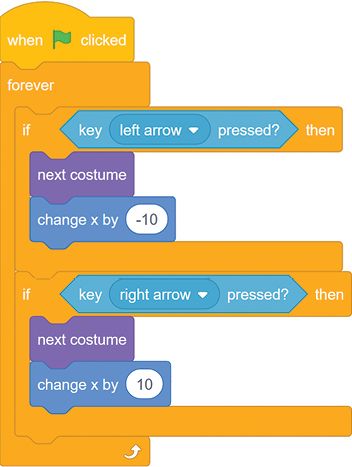
Inside the forever
loop, the program checks whether the left arrow or right arrow key is being pressed. If one of these arrows is pressed, the Cat
sprite switches to the next costume and changes its x position by -10
(moves to the left) or 10
(moves to the right). The Cat
sprite comes with two costumes that you can view by clicking the Costumes tab above the Block Palette. By switching between the two costumes using the next
costume
block, you can make it look like the cat is walking.

Make a Hovering Basketball Hoop
Now that the Cat
sprite is complete, let's move on to the next sprite in the game: the basketball hoop.
5. Create the Hoop Sprite
Click the Paint button, which appears after you tap or hover over the Choose a Sprite button. The drawing tool buttons will appear on the left side of the Paint Editor. Select a yellow color and use the Circle tool to draw a hoop. You can also change the width in the text field next to the Fill and Outline color boxes to make the circle's line thicker. Make sure the Paint Editor's crosshairs are in the center of the hoop.
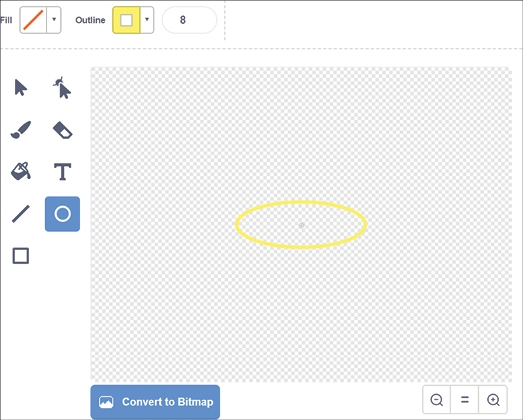
Rename the sprite Hoop
in its Sprite Pane. We want the Hoop
sprite to play a cheer sound when the player makes a basket, so let's load the Cheer
sound next. Click the Sounds tab at the top of the Block Palette and then click the Choose a Sound button in the lower left. When the Sound Library window opens, select Cheer
.
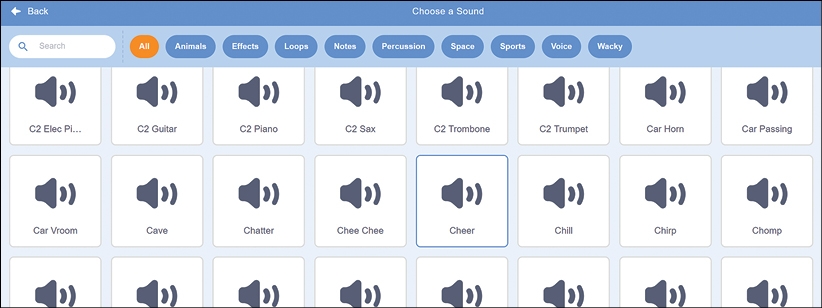
The Cheer
sound will now appear as an option for the start
sound
block you'll add to the Hoop
sprite.
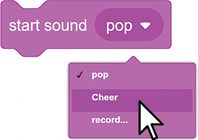
Add the following code to the Hoop
sprite to make it glide randomly around the top half of the Stage. You'll need to create a broadcast message by clicking the when
I
receive
block's white triangle and selecting new message. Name the new broadcast message swoosh
.
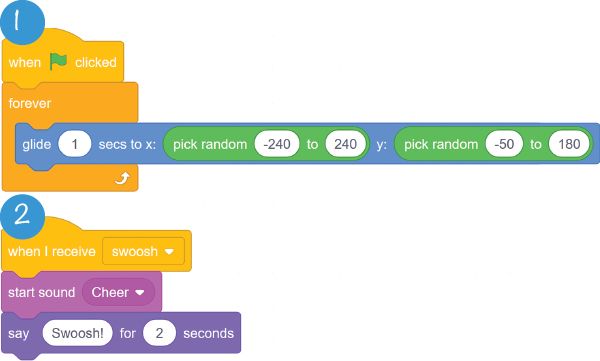
Script 1 makes the hoop slide to a new position every second. A moving hoop will make the game more challenging to play! Script 2 plays the Cheer
sound and displays "Swoosh!" when the swoosh
broadcast is received. Later, we'll make the Basketball
sprite broadcast this message when a basket is made.
6. Create the Hitbox Sprite
Now let's think about how to create code that determines whether the player makes a basket. We could write a program that checks whether the basketball is simply touching the hoop. But if we did that, just the edges of the basketball touching the hoop would count as a basket. We want the basket to count only if the basketball goes through the middle of the hoop. We'll have to think of a better fix.
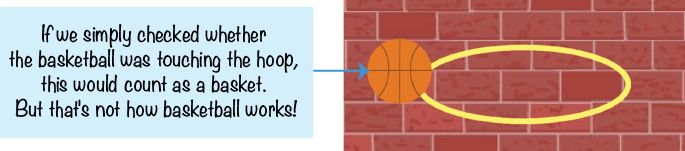
Instead, you can create a hitbox. A hitbox is a game-design term for a rectangular area that determines whether two game objects have collided with each other. We'll make a hitbox sprite. Create a new sprite by clicking the Paint button, which appears after you tap or hover over the Choose a Sprite button. Draw a small black square in the middle of the crosshairs by using the Rectangle tool and selecting the solid fill option. Rename this sprite Hitbox
. The Hitbox
sprite will look like this:
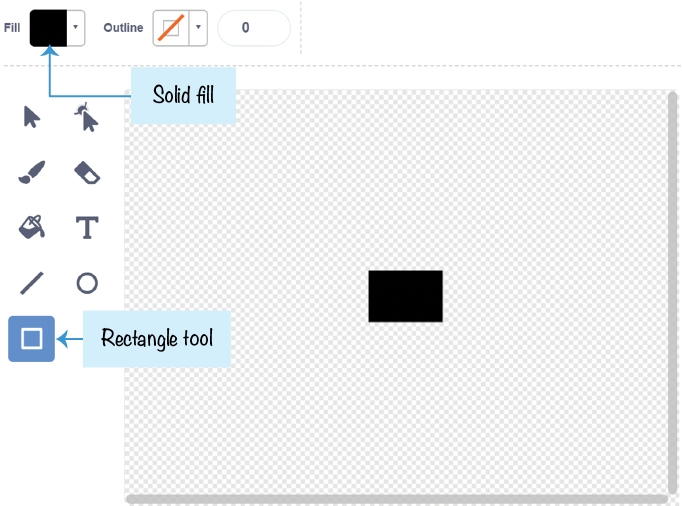
Add the following code to the Hitbox
sprite:
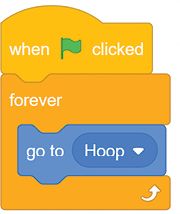
The Hitbox
sprite will now follow the Hoop
sprite, no matter where it glides.
In step 9, we'll write a program that makes sure a basket counts only if the basketball is touching the Hitbox
sprite, not the Hoop
sprite. The basketball will have to be much closer to the middle of the hoop to count as a basket!
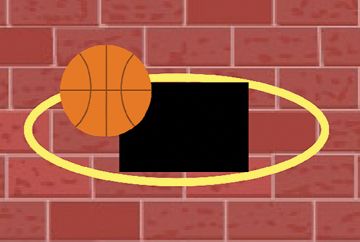
It looks odd to see a black square in the middle of the Hoop
sprite, so let's make the Hitbox
sprite invisible. Add the set
ghost
effect
block (it originally appears in the Block Palette as set color effect, but you can change "color" to "ghost") to the Hitbox
sprite and set it to 100
.
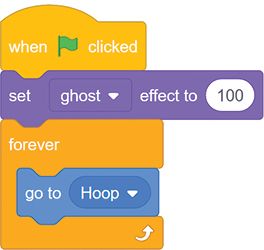
There's a difference between the hide
block and the set
ghost
effect
to
100
block. If you used the hide
block to make the Hitbox
sprite invisible, the touching blocks would never detect that the ball was touching the Hitbox
sprite, and the player would never be able to score. The set
ghost
effect
to
100
block makes the sprite invisible to you, but still allows the touching blocks to detect the Hitbox
sprite's presence.
Make the Cat Shoot Hoops
Next, you'll add a basketball for the cat to throw. Like the cat, the basketball will have gravity code and drop to the ground.
7. Create the Basketball Sprite
Click the Choose a Sprite button in the lower right to open the Sprite Library window. Select the Basketball sprite.
Next, click the Sounds tab at the top of the Block Palette. Then click the Choose a Sound button at the lower left to open the Sound Library window. Select the Pop
sound. Click the Scripts tab at the top of the Block Palette to bring back the Code Area.
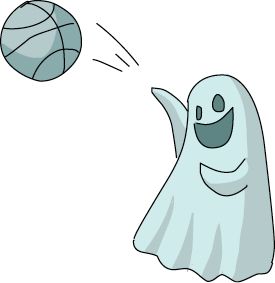
Now go to the orange Variables category. You'll make two variables. Click the Make a Variable button. Name the variable y
velocity
and make sure For this sprite only is selected before you click OK. Because they are For this sprite only variables, the Basketball
sprite's y
velocity
variable is separate from the Cat
sprite's y
velocity
variable. Even though they have the same name, they are two different variables.
Click the Make a Variable button again to make another variable named Player
1
Score
, but this time select For all sprites. (We capitalize Player
1
Score
because we'll make it visible on the Stage. Uncheck the box next to y
velocity
to hide it on the Stage.) The new variable blocks should appear in the orange Variables category.
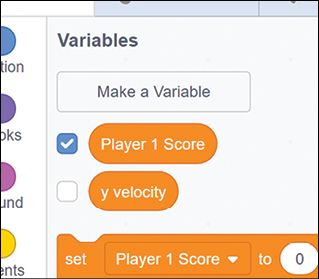
8. Add the Code for the Basketball Sprite
After you've added the pop
sound and two variables, add the following code to the Basketball
sprite.
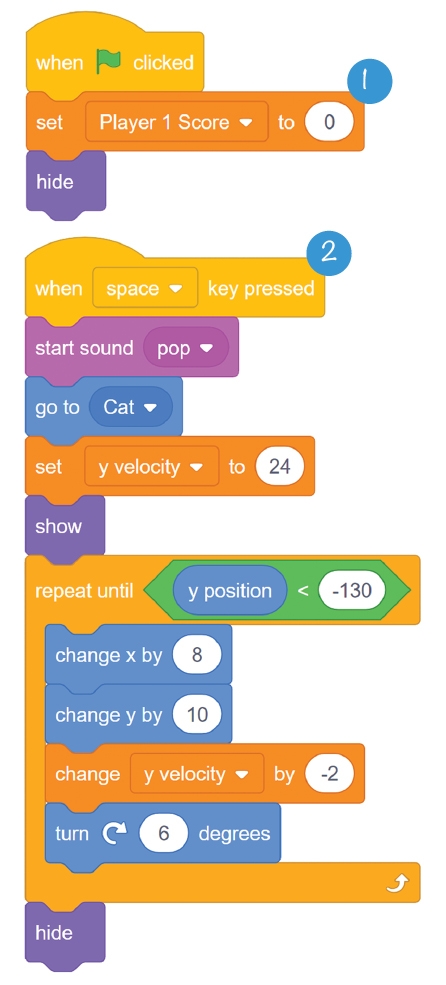
Script 1 makes sure the player starts with 0 points and hides the Basketball
sprite to start.
Script 2 uses code similar to the Cat
sprite code. When the player presses the spacebar, the basketball appears in front of the cat and starts moving forward. The code sets the Basketball
sprite's y
velocity
variable to a positive number, just like how the Cat
sprite's y
velocity
variable is set to a positive number when the cat jumps; this is how the cat throws the ball.
The repeat
until
y
position
<
-130
block will keep the Basketball
sprite falling until it reaches the ground. When it reaches the ground, the basketball will be hidden until the next time the player presses the spacebar.
9. Detect Whether a Basket Is Made
Next, you'll add the code that checks whether the Basketball
sprite is touching the Hitbox
sprite. This is how you know whether a basket has been made, and if it has, the Player
1
Score
variable should increase. However, there is one snag: the basket shouldn't count if the basketball goes up through the hoop.
Keep in mind that if the y
velocity
variable is positive, the change
y
by
y
velocity
block will move the Basketball
sprite up. If y
velocity
is 0
, then the Basketball
sprite is not moving up or down. But if y
velocity
is a negative number, then the Basketball
sprite is falling.
So, you'll add another if
then
condition to the Basketball
sprite's code. You'll increase the score only if the basketball is touching the hitbox (using the touching
Hitbox?
block) and moving downward (y
velocity
<
0
).
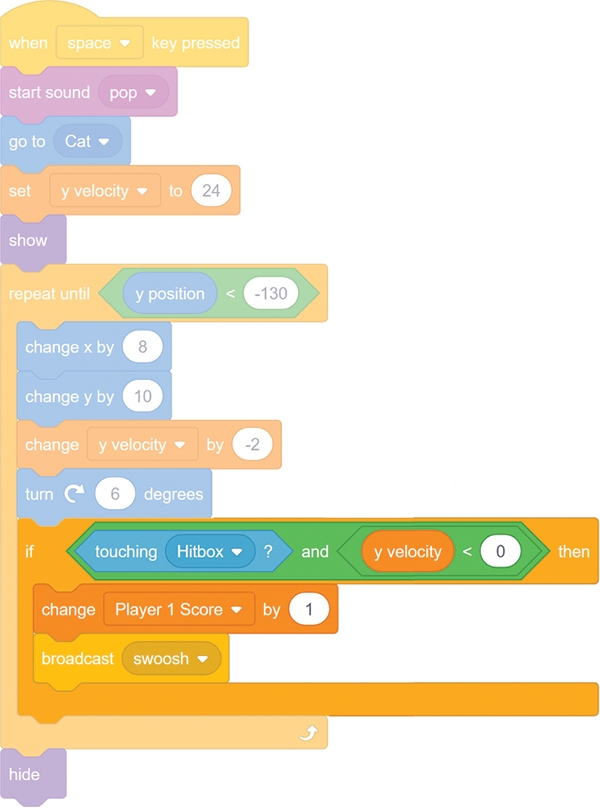
The and
block combines two conditions. For Scratch to run the code blocks inside the if
then
block, both of these conditions must be true. It isn't enough for the sprite to be touching the Hitbox
sprite or for the y
velocity
variable to be less than 0
. Both touching
Hitbox?
and y
velocity
<
0
must be true for the player to score. If these conditions are true, the Player
1
Score
variable is increased by 1
, and the swoosh
message is broadcast.
10. Fix the Scoring Bug
Did you notice that Player
1
Score
increases by several points for a single basket? This is a bug, which is a problem that makes the program behave in an unexpected way. We'll need to take a careful look at the code to figure out why this happens.
The repeat
until
loop block keeps looping until the ball hits the ground, so all of this code is for a single throw. The repeat
until
loop block checks several times whether the Basketball
sprite is touching the Hitbox
sprite and falling. The Player
1
Score
value should increase only the first time.
You fix this bug by creating a new variable that keeps track of the first time the basketball touches the hoop for a single throw. Then you can make sure the player scores a point only once per throw.
Click the orange Variables category in the Block Palette and then click Make a Variable. Name the variable made
basket
and select For this sprite only. Then modify the Basketball
sprite code.
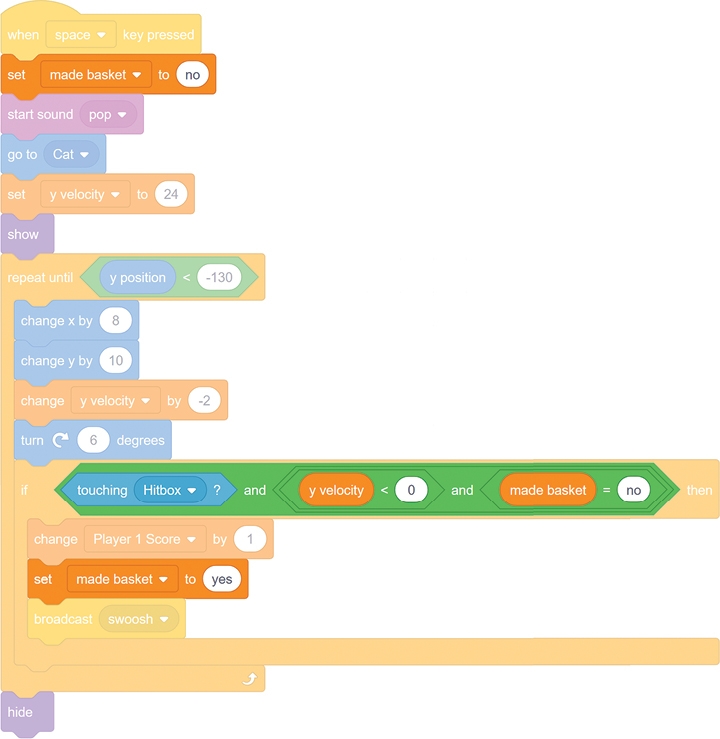
The made
basket
variable is set to no
when the player first presses the spacebar. This makes sense, because the player has not made a basket when the ball is initially thrown. We'll also use an and
block to add another condition to the code that checks whether a basket has been made. A basket is now detected, and the code in the if
then
block is run when three conditions are true:
- The
Basketball
sprite is touching theHitbox
sprite. - The
y
velocity
variable is negative (the basketball is falling). - The
made
basket
variable is set tono
.
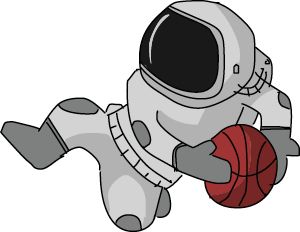
The first time the Basketball
sprite detects it has made a basket, it increases Player
1
Score
by 1 point and sets made
basket
to yes
. In future checks for that shot, made
basket
will not be equal to no
, so the basket is no longer detected. The made
basket
variable resets to no
the next time the player presses the spacebar to throw the basketball.
The Complete Program
Here is the final code. If your program isn't working correctly, check your code against this code.
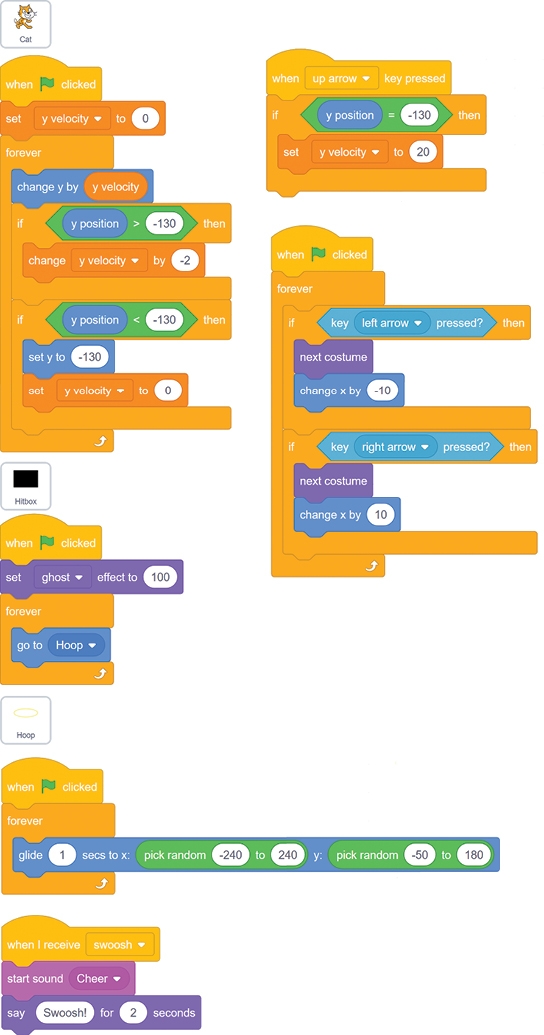
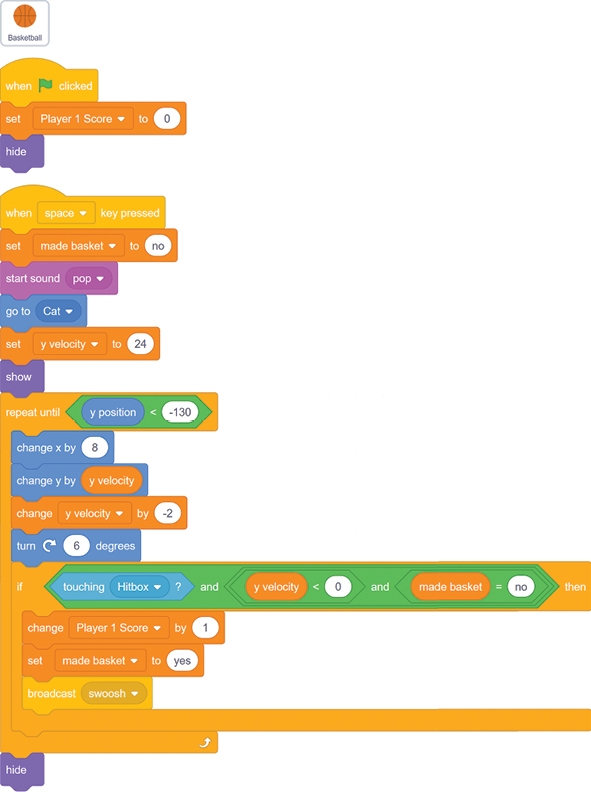
Cheat Mode: Freeze the Hoop
The moving basketball hoop is a hard target to hit. Let's add a cheat that will freeze it in place for a few seconds when a player presses the 7 key.
Select the Hoop
sprite and click the Make a Variable button to create a new For this sprite only variable named freeze
. Then modify the code for the Hoop
sprite to match the following code:
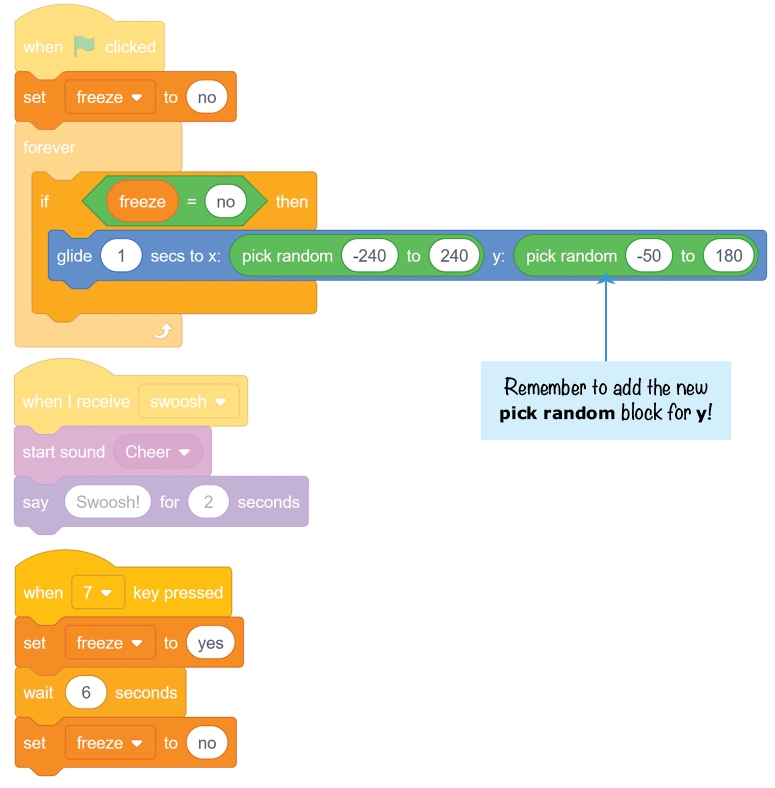
Summary
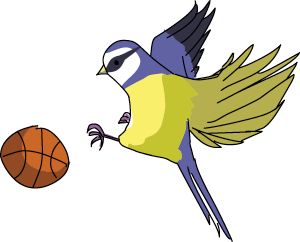
In this chapter, you built a game that
- Implements gravity and realistic falling
- Has a side-view perspective instead of a top-down view
- Uses variables to keep track of scores, falling speeds, and the first time a basket is made
- Has a hitbox to detect when a basket is made
The use of gravity in this program was pretty simple. By the time you reach Chapter 7, you'll be able to make an advanced platformer game with more complex jumping and falling. But there are plenty of Scratch programming techniques to practice first. In Chapter 5, you'll make a side-view game that uses cloning to duplicate a sprite dozens of times.
Source: https://inventwithscratch.com/book3/chapter4.html
0 Response to "How to Make Gravity in Scratch How to Make Gravity in Scratch Easy"
Post a Comment